In the ever-evolving world of software development, understanding the fundamentals of object-oriented programming (OOP) is crucial. This programming paradigm uses “objects” — components that bundle data and functionality together — to create more flexible, modular, and scalable software. By mimicking real-world interactions, OOP allows developers to craft solutions that are more intuitive and easier to debug, maintain, and enhance.
Fundamentals of Object Oriented Programming
What Is Object-Oriented Programming?
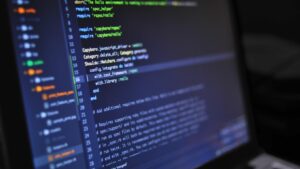
Object-oriented programming (OOP) stands as a programming paradigm that utilizes “objects” — data structures consisting of data fields and methods together. Programmers define the data type of a data structure, and the types of operations that can be applied to the data structure. In practice, OOP helps manage complexity by mimicking real-world behaviors, facilitating code that is more modular and predictable.
At its core, OOP focuses on the concepts of “objects” and “classes.” Objects are instances of classes, which collectively define the properties and methods that their instances can use. This approach contrasts significantly with procedural programming, which focuses on functions or procedures to manipulate data.
The Four Pillars of OOP
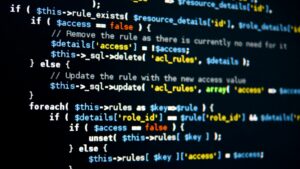
The four foundational principles of object-oriented programming are encapsulation, abstraction, inheritance, and polymorphism. These principles not only support the creation of complex software systems but also enhance the maintainability and flexibility of these systems.
- Encapsulation: Encapsulation involves bundling the data (variables) and the methods (functions) that work on the data into a single unit or class. It also restricts direct access to some of an object’s components, which can prevent the accidental modification of data.
- Abstraction: Abstraction simplifies complex reality by modeling classes appropriate to the problem, and working at the most relevant level of inheritance. It hides all but the relevant data about an object in order to reduce complexity and increase efficiency.
- Inheritance: Inheritance allows a new class to inherit the properties and methods of an existing class. This feature facilitates a hierarchical organization of classes and less redundancy of code. For instance, a “Vehicle” class might give way to subclasses like “Car” and “Motorcycle,” each inheriting traits from “Vehicle” while defining their own unique attributes.
- Polymorphism: Polymorphism gives a way to use a class exactly like its parent so there’s no confusion with mixing types. Yet, each child class keeps its own methods as needed to adapt a method of a parent class to perform a different task. For example, a “Draw” method in a “Shape” class could be adapted differently in “Circle” and “Rectangle” classes.
These principles form the backbone of object-oriented programming and are crucial for developers who create applications using languages like Java, C#, Python, and more. This foundational knowledge helps them build more robust, scalable, and maintainable software solutions.
Key Concepts in Object-Oriented Programming
Classes and Objects
Classes act as blueprints for creating instances known as objects, which are the fundamental building blocks in object-oriented programming. A class defines the structure and behaviors (methods) that its objects will possess, encapsulating both data (attributes) and functions that manipulate the data. For instance, in a class named Vehicle
, attributes might include make
, model
, and year
, while methods might involve operations like start()
and stop()
.
Encapsulation and Data Hiding
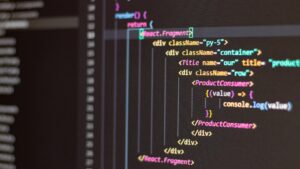
Encapsulation involves bundling the data (attributes) and the methods that modify the data into a single unit or class. This concept is closely tied with data hiding, wherein a class can hide its internal data to prevent outside interference and misuse. Data hiding is achieved using access modifiers like private
, protected
, and public
, which restrict the accessibility of components. For example, making an attribute private
in a class prevents it from being accessed directly from outside the class, thereby enforcing a controlled interaction through public methods.
Inheritance allows a new class, known as a child class, to adopt attributes and behaviors (methods) from another class, referred to as the parent class. This feature promotes code reusability and logical hierarchy. In programming languages like Java and C#, this is achieved using extends
or inherits
keywords. For example, a Car
class inheriting from the Vehicle
class immediately gains all non-private attributes and methods of the Vehicle
, which can then be further extended or modified.
Mastering the fundamentals of object-oriented programming is crucial for building robust and scalable software applications. Understanding key principles like encapsulation, inheritance, and polymorphism allows developers to create more maintainable and efficient code. Additionally, when developing applications that involve user data, integrating services from a customer data verification company can enhance the reliability and accuracy of your program’s data management, ensuring that the user information handled is both verified and secure.